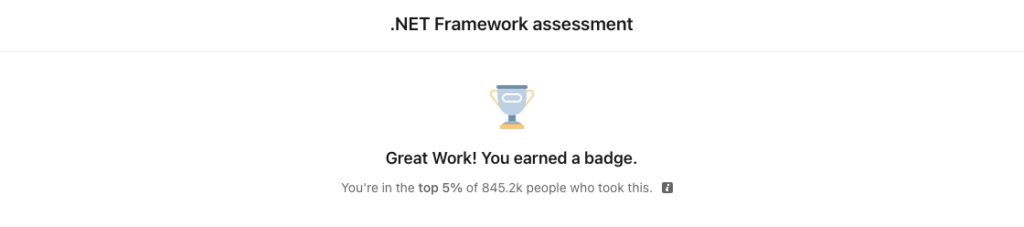
.net framework linkedin assessment answers
1. The ASP.NET Core Module is a native IIS module that plugs into the IIS pipeline to either _______.
- create IIS code the server needs in a file with the extension required, or run the IIS server in a mode compatible for ASP.NET Core
- host an ASP.NET Core app inside of the IIS worker process, called the out-of-process hosting model, or forward web requests to a backend
ASP.NET Core app running the Kestrel server, called the in-process hosting model - host an ASP.NET Core app inside of the IIS worker process, called the in-process hosting model, or forward web requests to a backend ASP.NET Core app running the Kestrel server, called the out-of-process hosting model
- package up your C# application and C# packages into .NET modules, or specify which of its packages should be visible to other .NET modules
Explanation:
The ASP.NET Core Module enables ASP.NET Core apps to run with IIS using either in-process hosting or out-of-process hosting (via Kestrel).
2. You want to encapsulate a command request as an object. Which design pattern best fits this objective?
- Command
- Observer
- Iterator
- Facade
Explanation:
The Command pattern encapsulates a request as an object, allowing parameterization of clients with different requests and support for undoable operations.
3. In the code below, what is the difference between RenderPartial and RenderAction?
@{
Html. RenderAction("Add");
Html. RenderPartial ("Add");
}
- RenderPartial will call an action method of the current controller and render a result inline. RenderAction will render the specified view inline without calling any action method.
- RenderPartial will call an action method of the current model and render a result inline. RenderAction will render the specified view inline without
calling any action method. - RenderAction will call an action method of the current model and render a result inline. RenderPartial will render the specified view inline without
calling any action method. - RenderAction will call an action method of the current controller and render a result inline. RenderPartial will render the specified view inline
without calling any action method.
Explanation:RenderPartial
is used to render a static partial view, while RenderAction
invokes a controller action and renders its result.
4. What is the difference between a heap and a stack?
- The heap is stored value types; the stack is stored reference types.
- The stack contains stored value types; the heap contains stored reference types.
- The stack is stored object types; the heap is stored class types.
- The heap is stored object types; the stack is stored class types.
Explanation:
The stack is used for value types and method execution, while the heap is used for reference types and dynamically allocated memory.
5. What is the Liskov substitution principle?
- Aclass should have only a single responsibility—that is, only changes to one part of the software’s specification should be able to affect the specification of the class.
- Many client-specific interfaces are better than one general-purpose interface.
- Obiects in a program should be replaceable with instances of their subtypes without altering the correctness of that program.
- Software entities should be open for extension, but closed for modification.
Explanation:
This principle ensures that derived classes can substitute for their base classes without affecting functionality.
6. What is an interface in .NET?
- An interface is responsible for keeping track of what is actually executing and where each executing thread is.
- An interface declares a contract or behavior that implementing classes require. It may declare only properties, methods, and events with no access modifiers. All the declared members must be implemented.
- An interface allows developers to create new classes that reuse, extend, and modify the behavior defined in other classes
- An interface provides a partial implementation for functionality and some abstract or virtual members that must be implemented by the
inheriting entities. It can declare fields too.
Explanation:
Interfaces define a contract without implementation, which implementing classes must adhere to.
7. How can you receive form data without a model binder in a controller action?
- public IFormResult ReceivedDataByRequest()
{string theName= Request.Forms/”theName” I:
return View() ;} - public IFormResult ReceivedDataByRequest()
{string theName= Request.Form| “theName”];
return View);} - public IActionResult ReceivedDataByRequest()
{string theName= Request.Form[“theName”] ;
return View() ;} - publie IActionResult ReceivedDataByRequest()
{string theName= Request.Forms[“theName”] ;
return view:}
Explanation:
The Request.Form
object is used to access form data directly in the absence of a model binder.
8. You want to create an instance of several families of classes. Which design pattern best fits this objective?
- Abstract Factory
- Decorator
- Bridge
- Singleton
Explanation:
The Abstract Factory pattern provides an interface for creating families of related objects without specifying their concrete classes.
9. You want to create a class of which only a single instance can exist. Which design pattern best fits this objective?
- Decorator
- Singleton
- Bridge
- Adapter
Explanation:
The Singleton pattern ensures a class has only one instance and provides a global point of access to it.
10. What does CIL stand for?
- Commonly Interpreted Language
- Common Intermediate Language
- C# Intermediate Language
- C# Interpreted Language
Explanation:
CIL is the intermediate code generated by .NET compilers, executed by the CLR.
11. What is the dependency inversion principle?
- Software entities should be open for extension, but closed for modification.
- A class should have only a single responsibility-that is, only changes to one part of the software’s specification should be able to affect the
specification of the class. - Objects in a program should be replaceable with instances of their subtypes without altering the correctness of that program
- Entities must depend on abstractions, not on concrete implementations
Explanation:
This principle reduces the dependency on concrete implementations by relying on abstractions.
12. What is a thread?
- a series of related tasks or methods that together turn inputs into outputs
- a program that is running on your computer
- the basic unit to which an operating system allocates processor time
- a single operation that does not return a value and that usually executes asynchronously
Explanation:
A thread is the smallest unit of execution within a process.
13. What is a tuple?
- a group of classes designed specifically for grouping together objects and performing tasks on them
- a data structure that has a specific number and sequence of elements
- an object to store multiple variables of the same type in an array data structure
- an array whose elements are jagged
Explanation:
Tuples group multiple elements into a single object, maintaining order and size.
14. Which statement about a read-only variable is not true?
- It can be initialized at declaration onlv.
- At run time, its value is evaluated
- It can be initialized in either the constructor or the declaration
- It can be either static or an instance member.
Explanation:
A read-only variable can be initialized at declaration or in the constructor.
15. What is a namespace?
- a group of generic collections-in a logical hierarchy by function–that enable you to access the core functionality you need in your applications
- a group of classes, structures, interfaces, enumerations, and delegates-organized in a logical hierarchy by function- that enable you to access the core functionality you need in your applications
- a group of methods-in a logical hierarchy by class–that enable you to access the core functionality you need in .NET
- a group of assemblies-in a logical hierarchy by function-that enable you to access the core functionality you need in your applications
Explanation:
Namespaces organize related .NET components logically to prevent name conflicts and improve readability.