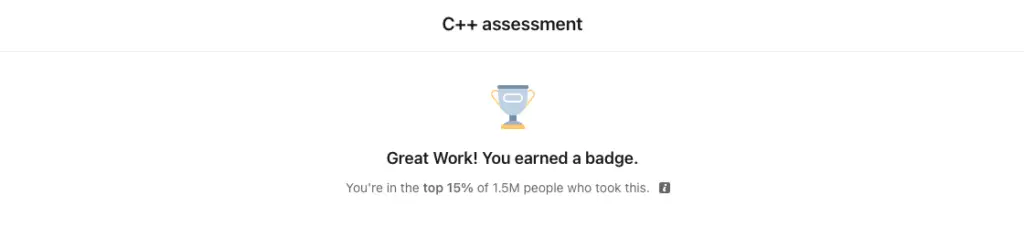
c++ linkedin assessment answers
1. What is the .* operator and what does it do?
- It is a combination of the member access operator (.) and the dereference operator (*), so it allows you to access the object that a member pointer points to.
- It is the member access with address-of operator, which returns the address of a class or struct member.
- It is the same as the class member access operator, or arrow operator (->), which allows you to access a member of an object through a pointer to the object.
- It is the pointer to member operator, and it allows you to access a member of an object through a pointer to that specific class member.
3. What is the result from executing this code snippet?
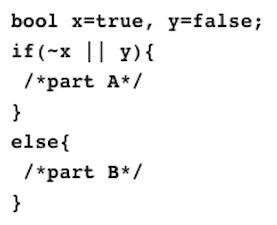
- Part A executes because the expression (~ | | y) always results in true if y==false.
- Part A executes because -x is not zero, meaning true.
- Part B executes because -x is false and y is false, thus the OR operation evaluates as false.
- Part B executes because the statement (~ y) is invalid, thus false.
4. What is a default constructor?
- a constructor that can be used with no arguments
- a constructor that does not have a return value
- a constructor that is used by multiple classes
- a constructor that initializes all members of a class
5. What is an include guard?
- a preprocessor statement that prevents a source file from being included more than once in a project
- a compiler option that prevents the user code from including additional libraries
- a library that adds safety features such as mutexes, watchdog timers, and assertions to the project
- a preprocessor directive that prevents inconsistent behaviors in lines that contain the #ifdef, #ifndef, or #elif directives
7. What will happen if you attempt to call this function with checkConcatThreshold("a"):?

- No compilation errors will occur and no compilation warnings will occur.
- A compilation error will occur.
- A compilation warning will occur and the second argument will be
given a default value of b. - A compilation warning will occur and the second argument will be given a default value of empty string.
8. A class destructor can be called when a variety of situations occur. Which choice is not one of those situations?
- The garbage collector detects that an object is no longer going to be used.
- The program is terminated. This calls the destructor of static duration objects.
- The delete () function is called for an object pointer assigned with the new operator.
- An automatic storage duration object goes out of scope.
9. When placed in a valid execution context, which statement will dynamically allocate memory from the heap for an integer of value 11?
- int anInt = new int(11);
- int* anInt = new int[11];
- int anInt = new int[11];
- int* anInt = new int (11) ;
10. What is the output of this piece of code?
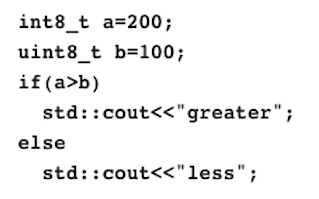
- There is no output because there is a compiler error.
- greater
- less
- There is no output because there is an exception when comparing an int8 t with a uint&t.
11. Which choice does not produce the same output as this code snippet? Assume the variable i will not be used anywhere else in the code
for(i=l:i<10:it+)
cout<<i<<endl;
- i=1:
do{
cout<<i++<<endl;
}while(i<10); - i=1;
loop:
cout<<i++<<endl;
if (i<10) goto loop; - for (int i:{1,2,3,4,5,6,7,8,9})
cout<<i<<endl; - i=1;
while(i<10){
cout<<++i<<endl;
}
13. What would be the output of this code?

- 0
- 243
- The output is the addresses of nums [ 0 ], nums|1], and nums [ 2],in that order, with no spaces.
- 256
14. Which choice best describes the type long?
- a 64-bit floating point number
- a string with more than 255 characters
- an integer number of at least 32 bits
- a pointer
15. What is the output of this piece of code?

- a=-56, b=100
- a=-55, b=100
- a=200, b=-156
- a=200, b=100