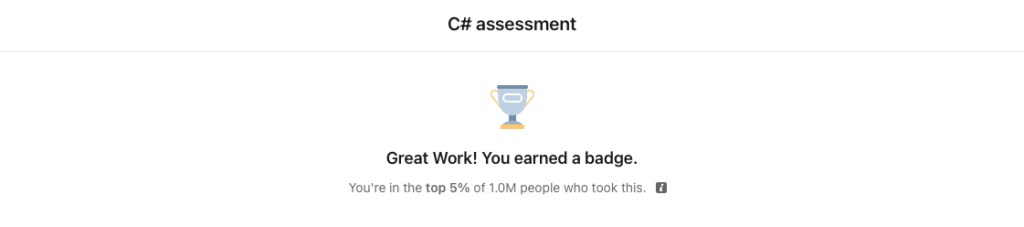
c# linkedin assessment answers
1. What is the correct way to write an event named apiResult based on a delegate named ResultCallback?
- public event ResultCallback( () -> apiResult);
- public event void Resultallback;
- public void event Resultallback apiResult;
- public event ResultCallback apiResult;
2. What kind of values can arrays store?
- class and struct instances
- multiple variables, or collections, of the same type
- unordered collections of numeric values
- key-value pairs of any C# supported type
3. What is the difference between the ref and out keywords?
- Variables passed to out specify that the parameter is an output parameter, while ref specifies that a variable may be passed to a function without being initialized.
- Variables passed to out can be passed to a function without being initialized, while ref specifies that the value is a reference value that can be changed inside the calling method.
- Variables passed to ref can be passed to a function without being initialized, while out specifies that the value is a reference value that can be changed inside the calling method.
- Variables passed to ref specify that the parameter is an output parameter, while out specifies that a variable may be passed to a function without being initialized.
4. What character would you use to start a regular expression pattern at a word boundarv?
- \a
- \w
- d
- \b
5. How would you determine if a class has a particular attribute?
- Attribute.GetCustomAttribute(typeof(ExampleControlle typeof(SubControllerActionToViewDataAttribute))
- Attribute.GetCustomAttribute, typeof(SubControllerActionToViewDataAttribute)
- var type = typeof (SomeType) ; var attribute = type.GetCustomAttribute<SomeAttribute>0):
- typeof (MyPresentationModel) .Should ( ) .BeDecoratedwith () ;
6. What are the four keywords associated with exception handling in C#?
- try, catch, finally, throw
- try, valid, finally, throw
- try, catch, invalid, valid
- finally, throw, invalid, valid
7. How do you indicate that a string might be null?
- string (nullable) myVariable
- string? myvariable
- A string cannot be nullable.
- string myVariable = null
8. When an asynchronous method is executed, the code runs but nothing happens other than a compiler warning. What is most likely causing the method to not return anything?
- The yield keyword is missing from the method.
- The method is missing an await keyword in its body.
- The wait keyword is missing from the end of the method.
- The return yield statement is missing at the end of the method.
9. In which of these situations are interfaces better than abstract classes?
- You should use both an interface and an abstract class when defining any complex object.
- Interfaces are a legacy of older versions of C# and are interchangeable with the newer abstract class feature.
- When you need a list of capabilities and data that are classes-agnostic, use an interface. When you need a certain object type to share characteristics, use an abstract class.
- When you need to define an object type’s characteristics, use an interface. When you need to define an object type’s capabilities, use an abstract class.
10. How would you access the last two people in an array named People?
- You cannot do this in C#.
- People[^2]
- People.[..^2]
- People[..^3]
11. What is the difference between a. Equals (b) and a == b?
- The .Equals method compares primitive values while == compares all values.
- The Equals method compares reference identities while the == compares contents.
- The . Equals method compares reference types while == compares primitive value types.
- The .Equals method compares contents while == compares reference identity.
12. Do you need to declare an out variable before you use it?
- No, out variables are no longer part of C#.
- No, you can declare an out variable in the parameter list.
- Yes.
- Yes, vou must declare an out variable if it is a primitive type.
13. Do you need to declare an out variable before you use it? user ID with a public get and private set?
- public int userID [get, private set] ;
- public int userID { get; private set; }
- public int userID <get, set>;
- public int userID = { public get, private set};
14. What is delegate?
- a custom variable type that can be used in abstract classes
- a type that holds a reference to a method with a particular parameter list and return type
- a specific value type that can be used only in callback methods
- a variable that holds a reference to a value type and its content
15. How would you declare a sealed class named User?
- abstract User {}
- public sealed class User {}
- sealed class User {}
- private class User {}