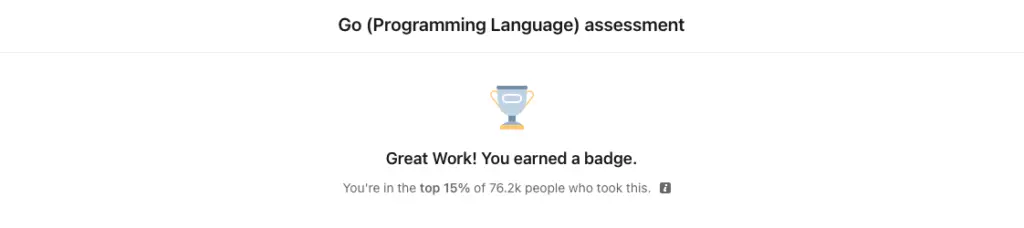
go programming language linkedin assessment answers
1. How would you signal to the Go compiler that the Namespace struct must implement the JSONConverter interface? This question assumes the answer would be included in the same package where Namespace is declared.
- type Namespace struct {
JSONConverter
// The rest of the struct declaration goes here } - var _ JSONConverter = nil. (*Namespace)
- var _ JSONConverter = (*Namespace) (nil)
- type Namespace struct {
implements JSONConverter
// The rest of the struct declaration goes here }
2. How can you compile main.go to an executable that will run on OSX arm64?
- Set GOOS to darwin and GOARCH to arm64.
- Set GOOS to arm64 and GOARCH to darwin.
- Set GOOS to osx and GOARCH to arm64.
- Set GOOS to arm64 and GOARCH to osx.
3. If you iterate over a map in a for range loop, in which order will the key:value pairs be accessed?
- in reverse order of how they were added, last in first out
- sorted by key in ascending order
- in pseudo-random order that cannot be predicted
- in the order they were added, first in first out
4. Which types can Go developers define methods for?
- all types
- all named types not built-in to Go, such as type Example int but not int, type Example struct{…} but not struct, etc.
- only types named struct, such as type Example struct{…}
- only types named struct, map, and slice, such as type Example struct{…}
5. What is the common way to have several executables in your project?
- Have a cm directory and a directory per executable inside it.
- Use build tags.
- Comment out main.
- Have a pkg directory and a directory per executable inside it.
7. Given the definition of worker below, what is the right syntax to start a start a goroutine that will call worker and send the result to a channel named ch?
func worker (m Message) Result
8. How can you avoid a goroutine leak in this code?

- Use a sync.WaitGroup.
- Make ch a buffered channel.
- Add a default case to the select.
- Use runtime.SetFinalizer.
9. Which encodings can you put in a string?
- any Unicode format
- any, it accepts arbitrary bytes
- UTF-8
- UTF-8 or ASCII
10. How could you recover from a panic () thrown by a called function without allowing your program to fail assuming your answer will run in the same scope where your function call will experience the panic?
- Prefix the function call with e to force it to return the panic as an error value and then handle the error just as you would an error returned by any function.
- Wrap the function call in an anonymous function with a return type of panic, remembering to invoke the anonymous function by suffixing it with (), then introspecting the returned panic instance to handle the error.
- Use try{…} to wrap the code calling the function and then handle the error within the catch{ … }.
- Use defer func() {…} before the function call with the error and then handle the panic inside the anonymous function.
11. Which is a valid Go time format literal?
- “year-month-day”
- “YYYY-mm-dd”
- “2006-01-02”
- “y-mo-d”
12. How can you view the profiler output in cpu.pprof in the browser?
- go tool trace cpu.pprof
- go tool pprof -http=: 8080 cpu.pprof
- go tool prof cpu.pprof
- go pprof -to SVG cpu.prof
13. According to the Go documentation standard, how should you document this function?
func Add (a, b int) int {
return a + b
}
14. What does the built-in generate command of the Go compiler do?
- It scans the project’s source code looking for //go: generate comments, and for each such comment runs the terminal command it specifies.
- It looks for files with names that end with generate. go, and then compiles and runs each of these files individuallv.
- It has subcommands mocks and tests to generate relevant . go source files.
- It provides subcommands sql, json, yaml, and switches — schema and –objects to generate relevant code.
15. What would happen if you attempted to compile and run this Go program?
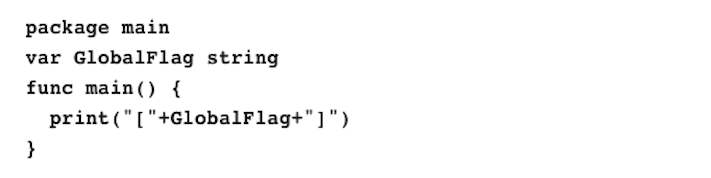
- It would not compile because GlobalFlag was never initialized.
- It would compile and print nothing because “[“+nil+”)” is also nil.
- It would compile and print []
- It would compile but then panic because GlobalFlag was never initialized.