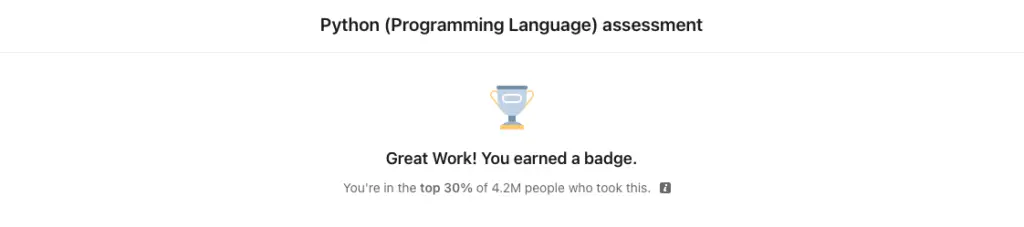
python linkedin assessment answers
1. NumPy allows you to multiply two arrays without a for loop. This is an example of ______.
- functional programming
- attribution
- vectorization
- acceleration
2. Given a list defined as numbers = 1,2,3,41, what is the value of numbers[-2]?
- 3
- 2
- An IndexError exception is thrown.
- 1
3. In this code fragment, what will the values of c and d be equivalent to?
import numpy as np
a = np.array ([1,2,3])
b = np.array ([4,5,6])
c = a*b
d = np.dot (a, b)
4. In Python, how can the compiler identify the inner block of a for loop?
- because of the end keyword at the end of the for loop
- because the block is surrounded by brackets ({})
- because of the level of indentation after the for loop
- because of a blank space at the end of the body of the for loop
5. What will be the value of x after running this code?
x = {1, 2, 3, 4, 5}
x.add(5)
x.add(6)
- {5, 6, 1, 2, 3, 4, 5}
- {6, 1, 2, 3, 4, 5}
- {1, 2, 3, 4, 5, 6}
- {1, 2, 3, 4, 5, 5, 6}
6. Jaccard Similarity is a formula that tells you how similar two sets are. It is defined as the cardinality of the intersection divided by the cardinality of the union. Which choice is an accurate implementation in Python?
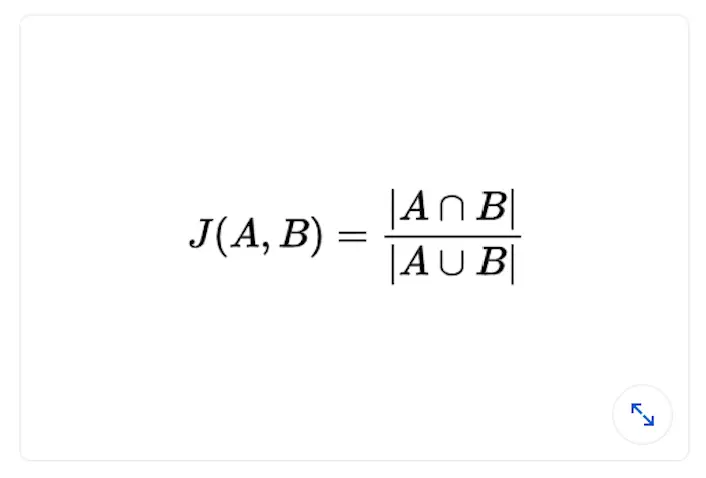
- def jaccard(a, b): return a. intersection (b) / a. union(b)
- def jaccard(a, b): return len (a | b) / len ( a & b )
- def jaccard(a, b): return len (a & b) / len ( a | b )
- def jaccard(a, b): return len (a && b) / len ( a ll
b )
7. Suppose you want to double-check if two matrices can be multiplied using NumPy for debugging purposes. How would you complete this code fragment by filling in the blanks with the appropriate variables?
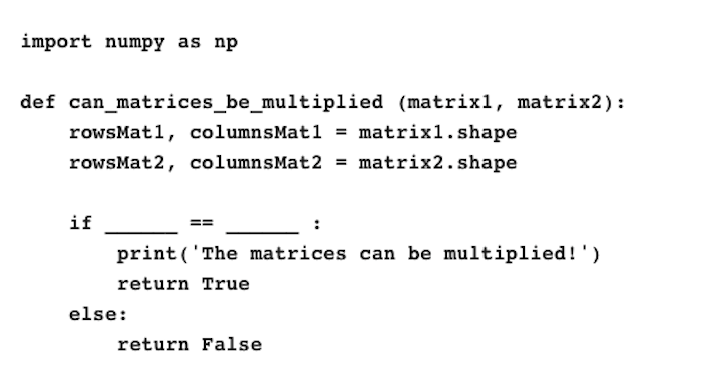
- columnsMat1; rowsMat2
- columnsMat1; rowsMat1;
- columnsMat2; rowsMat1
- columnsMat1; columnsMat2
8. What will this code print?
number = 3
print (f"The number is {number}")
- the number is 3
- It throws a TypeError because the integer must be cast to a string.
- The number is 3
- THE NUMBER IS 3
9. What is the output of this code? (NumPy has been imported as np.)
a = np. array ([1,2,3,4])
print (a[[False, True, False, False]])
- [0,2,0,0]
- [2]
- {2}
- {0,2}
10. What will this line of code return? (Assume n is already defined as any positive integer value.)
[ x*2 for x in range (1,n) ]
- a list with all the even numbers less than 2*n
- a list with all the even numbers less than or equal to 2*n
- a list with all the odd numbers less than 2*n
- a dictionary with all the even numbers less than 2*n
11. Which fragment of code will print exactly the same output as this fragment?
import math
print (math.pow(2,10)) # prints 2 elevated to the 10th
power
12. When an array is large, NumPy will not print the entire array when given to the built-in print function. What function can you use within NumPy to force it to print the entire array?
- set_printoptions
- set_fullprint
- set_printparams
- set_printwhole
13. Using Pandas, we load a data set from Kaggle, as structured in the image below. Which command will return the total number of survivors?
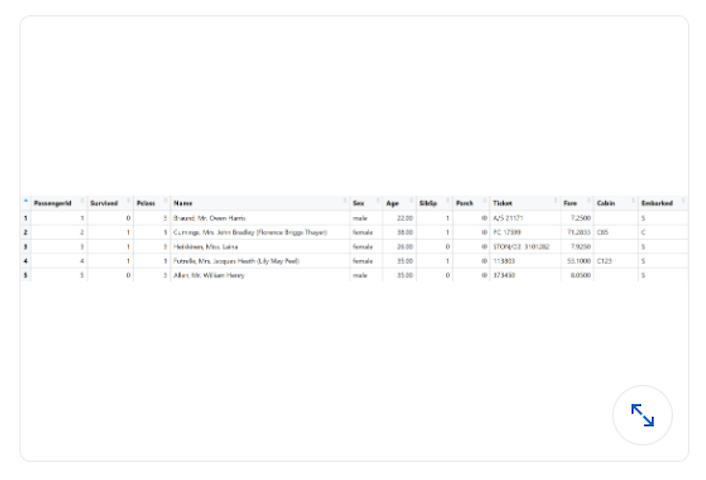
- sum(titanic[ ‘Survived’ ]==0)
- [x for x in titanic[ ‘Survived’ ] if x == 1]
- len(titanic[ “Survived” ])
- sum(titanic[ ‘Survived’ ])
14. What two functions within the NumPy library could you use to solve a system of linear equations?
- linalg.inv() and .eye()
- linalg.det () and . dot( )
- linalg.inv() and .dot()
- linalg.eig() and .matmul()
15. What will this statement return?
{x : x*x for x in range (1,100) }
- a list with all numbers squared; from 1 to 99
- a set of tuples, consisting of (x, x squared); from 1 to 99
- a dictionary with x as a key, and x squared as its value: from 1 to 100
- a dictionary with x as a key, and x squared as its value; from 1 to 99