advanced react coursera week 4 quiz answers
Final graded quiz: Advanced React
1. You are building a form using both Formik and Yup libraries, where one of the inputs is an email. Before the form gets submitted to the server, you would like to set up some client validation with Yup to make sure the field has an email that is valid, otherwise a message “Invalid email address” would be shown on the screen. This field is also required. Choose the correct validation code from the three code snippets.
- Yup.email().string(“Invalid email address”).required(“Required”)
- Yup.email(“Invalid email address”).required(“Required”)
- Yup.string().email(“Invalid email address”).required(“Required”)
2. You have the following React application where you have a ToDo component that has two text labels and an uncontrolled text input and the entry point App component that renders a list of two ToDos and a button to reverse the order of the ToDos. To avoid a React keys warning, a key is provided to each ToDo component, with the index as its value. Suppose that the next sequence of events happen in the application:
1. You write “Wash dishes” in the first ToDo input
2. You write “Buy groceries” in the second ToDo input
3. You click the button to reverse the order
What would happen on the screen after that?
const ToDo = props => (
< tr>
< td>
< label>{props.id}< /label>
< /td>
< td>
< input />
< /td>
< td>
< label>{props.createdAt}< /label>
< /td>
< /tr>
);
function App() {
const [todos, setTodos] = useState([
{
id: 'todo1',
createdAt: '18:00',
},
{
id: 'todo2',
createdAt: '20:30',
}
]);
const reverseOrder = () => {
// Reverse is a mutative operation, so we need to create a new array
first.
setTodos([...todos].reverse());
};
return (
< div>
< button onClick={reverseOrder}>Reverse< /button>
{todos.map((todo, index) => (
))}
< /div>
);
}
< /div>
);
}
todo2 Buy groceries 20:30
todo1 Wash dishes 18:00
todo2 Wash dishes 20:30
todo1 Buy groceries 18:00
todo1 Buy groceries 18:00
todo2 Wash dishes 20:30
3. A team is tasked with implementing a ThemeProvider for an application that will inject into the tree a light/dark theme, as well as a toggler function to be able to change it. For that, the following solution code has been incorporated. However, unwittingly, some errors have been introduced that prevent it from working correctly. What are the errors in the code solution? Select all that apply.
import{ createContext, useContext, useState} from"react";
const ThemeContext = createContext(undefined);
export const ThemeProvider= () => {
const[theme, setTheme] = useState("light");
return(
< ThemeContext.Provider
value={{
theme,
toggleTheme: () => setTheme(!theme),
}}
>
< /ThemeContext.Provider>
);
};
- The default value of createContext should be “light” instead of undefined.
- The children are not passed through
- There is no need to use local state for the context.
- The toggleTheme implementation is incorrect.
4. True or False: The type of a React element can be a DOM node, such as, for example, an HTML button.
- True
- False.
5. Assuming you have the following set of components, what would be logged into the console when clicking the Submit button that gets rendered on the screen?
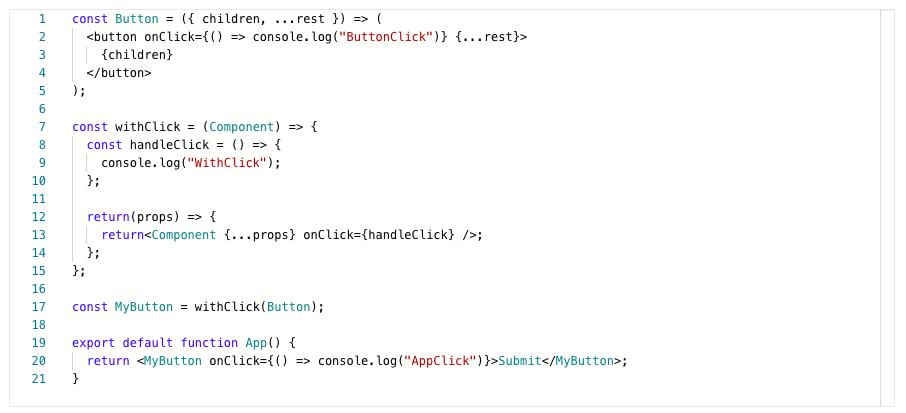
- “ButtonClick”.
- “WithClick”
- “AppClick”
6. True or False: Using jest and react-testing-library, to assert that a function has been called with some specific arguments, you would need to use the toHaveBeenCalledWith matcher.
- False.
- True.
7. True or false: The following piece of code is an example of the render props pattern.
< LoginUser renderUser={< p>Mark} />
- True
- False
8. You need the below code snippet to run only after the initial render. What updates (if any) do you need to make to the code?
React.useEffect(()=> {
console.log('The value of the toggle variable is', toggle)
})
- Add an empty dependency array.
- You shouldn’t make any updates.
- You should remove the toggle variable.
9. True or false? In the following component, the setRestaurantName variable’s value will not be reset between re-renders of the App component.

- True
- False
10. Is the following code snippet valid? Why?
if (data !== '') {
useEffect(() => {
setData('test data');
});
}
- It’s not valid, because it’s breaking the rules of hooks.
- It’s valid, because it’s not breaking the rules of hooks.
- It’s valid, because you can use the useEffect() call in an if statement.