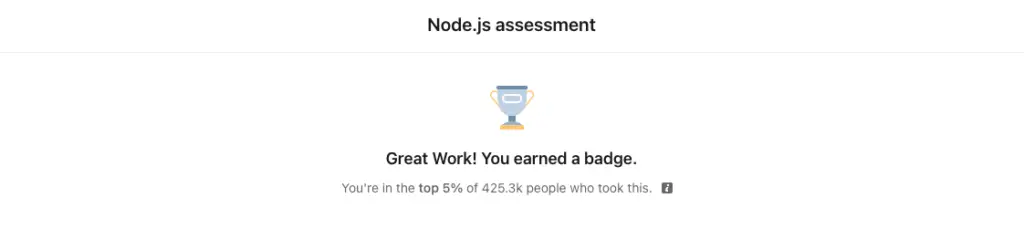
node.js linkedin assessment answers
1. Which assert module method is usually used to test the error-first argument in callbacks?
- fail
- ifError
- doesNotThrow
- deepStrictEqual
2. If the child process module methods are in scope, what is a correct way to execute the command ps -ef using a child process?
- fork( “ps -ef”)
- exec ( “ps -ef”)
- exec(“ps”, “-ef”)
- spawn (“ps -ef”)
3. When exploring the Node documentation's features, what are the stability ratings?
- They are a Node command to validate stability of your code
- They tell if a feature is LTS (Long Term Supported).
- They tell if a feature is ES6 compliant.
- They are an indication of the stability of Node.js modules and usage recommendations.
4. How can ECMAScript modules be used natively in Node?
- ECMAScript modules cannot be used natively in Node
- ECMAScript modules can be used natively in Node only by using a compiler like Babel.
- ECMAScript modules can be used natively in Node only by using a bundle like webpack.
- ECMAScript modules can be used natively in Node with the .mjs file extension.
5. When a request event is received in the HTTP module, what is the type of the first argument passed to that event, usually named req?
- http.ServerResponse
- http.ServerRequest
- http.IncomingMessage
- http.ClientRequest
6. If EventEmitter is in scope, which line of code will have an event emitter emitting a change event?
- new EventEmitter( ‘change’) ;
- EventEmitter.new().emit( ‘change’) ;
- EventEmitter.emit( ‘change’ ) ;
- (new EventEmitter ( )).emit( ‘change’ ) ;
7. How does it affect the performance of a web application when an execution path contains a CPU-heavy operation, such as calculating a long Fibonacci sequence?
- As Node.js is asynchronous, this is handled by a libuv and a threadpool. The performance will not notably degrade.
- As Node.js is asynchronous, this is handled by a threadpool and the performance will not notably degrade.
- As the application code runs asynchronously within a single thread, the execution will block, accepting no more requests until the operation is completed.
- The current thread will block until the execution is completed and the operating system will spawn new threads to handle incoming requests. This can exhaust the number of allowed threads (255) and degrade performance over time.
10. How would you determine the number of cluster instances to start when using the cluster module?
- const numInstances = require(‘os’ ).cpus () .length;
- const numInstaces = process.cpus () .length;
- const numInstances = cluster. instances () ;
- const numInstances = cluster.instances () .length;
11. You have a script.js file with the single line of code shown here. What will be the output of executing script.js with the node command?
console.log (arguments) ;
- an object representing an array that has five elements
- ReferenceError: arguments is not defined
- undefined
- an empty string
12. What is the importance of having good practices around status code in your response?
- It indicates success or failure to the client and helps with testing.
- It contains information about the current performance of the server.
- It is not important to have good practices regarding status codes.
- Response codes are the only way you can tell what is happening on the server.
14. Which statement about event emitters is false?
- Any values returned by the listeners for an emitted event are ignored.
- Event names must be camelCase strings.
- The emit method allows an arbitrary set of arguments to be passed to the listener functions.
- When an event emitter object emits an event, all of the functions attached to that specific event are called synchronously
15. Which file does node-gyp use to read the build configuration of a module?
- gyp.json
- package.gyp
- binding.gyp
- gypre