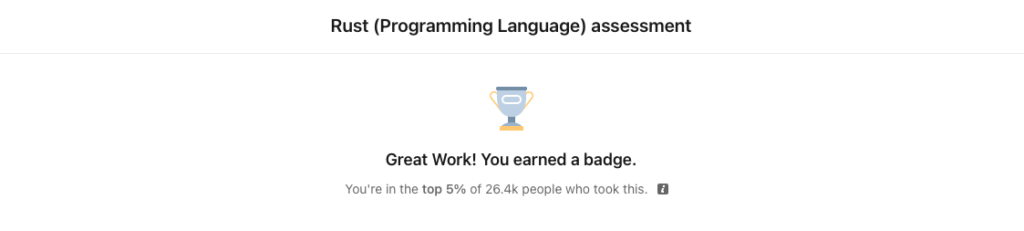
rust programming language linkedin assessment answers
1. The smart pointers Rc and Arc provide reference counting. What is the API for incrementing a reference count?
- .clone()
- .incr()
- .increment()
- .add()
2. Which fragment does not incur memory allocations while writing to a "file" (represented by a vec)?
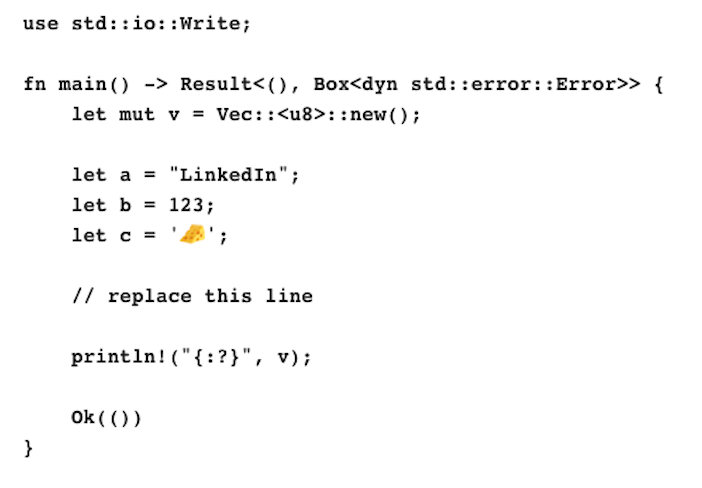
3. To convert a Result to an Option, which method should you use?
- .ok()
- .to_option()
- .into()
- .as_option()
4. Why does this code not compile?

- Closures are types and can be returned only if the concrete trait is implemented.
- The returned fn pointer and value need to be represented by another trait.
- Closures are types, so they cannot be returned directly from a function.
- Closures are represented by traits, so they cannot be a return type.
5. What smart pointer is used to allow multiple ownership of a value in various threads?
- Arc<T>
- Both Arc<r> and Rc<T> are multithread safe.
- Box<T>
- Rc<T>
7. Which statement about this code is true?
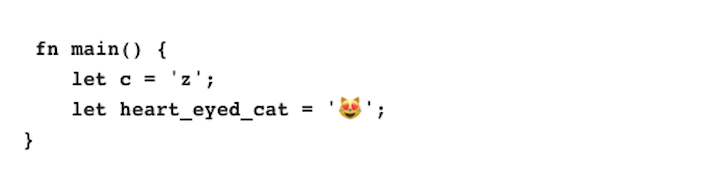
- Both are string literals.
- heart_eyed_cat is an invalid expression.
- c is a string literal and heart_eyed_cat is a character literal.
- Both are character literals.
8. Which types are not allowed within an enum variant's body?
- trait objects
- zero-sized types
- floating-point numbers
- structs
9. Which type cast preserves the mathematical value in all cases?
- i64 as i32
- i32 as i64
- f64 as f32
- usize as u64
10. Your application requires a single copy of some data tvpe T to be held in memory that can be accessed by multiple threads. What is the thread- safe wrapper type?
- Rc<Mutex<T>>
- Mutex<Arc<T>>
- Arc<Mutex<T>>
- Mutex<Re<T>>
12. Which is valid syntax for defining an array of 132 values?
- [i32; 10]
- Array<i32>:: new(10)
- [i32]
- Array<i32>: :with_capacity(10)
13. What is the effect of marking a function as pub (crate)?
- It is visible to other functions within the function’s module and any of its submodules.
- There is no effect. It is equivalent to the default.
- It is visible to members of all modules within the function’s crate.
- It is visible to code that is packaged as a crate.
15. Which cargo command checks a program for errors without creating a binary executable?
- cargo –version
- cargo init
- cargo check
- cargo build