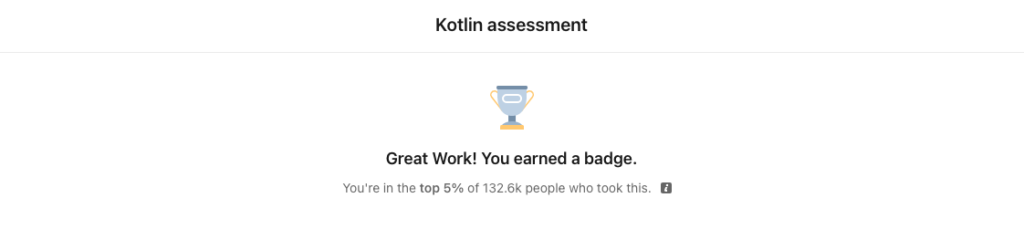
kotlin linkedin assessment answers
1. Which snippet correctly shows setting the variable max to whichever variable holds the greatest value, a or b, using idiomatic Kotlin?
- val max3 = a.max(b)
- val max = if (a > b) a else b
- if(a > b) max = a else max = b
- val max = a > b ? a : b
2. This code is occasionally throwing a null pointer exception (NPE). How can you change the code so it never throws an NPE?
println("length of First Name =
${firstName! !.length}")
- Replace !!. with ?..
- Surround the line with a try/catch block.
- Replace !!. with ? :.
- Replace !!. with ?.let.
3. How do you fill in the blank below to display all of the even numbers from 1 to 10 with the least amount of code?
for(______) {
println ("There are Scount butterflies.")
}
- var count=2; count <= 10; count+=2
- count in 1..10
- count in 1..10 & 2
- count in 2.. 10 step 2
4. Which line of code shows how to call a Fibonacci function, bypass the first three elements, grab the next six, and sort the elements in descending order?
- val sorted = fibonacci( ).skip(3).limit (6) .sortedByDescending ().toList ()
- val sorted = fibonacci( ).skip(3). take (6). sortedDescending().toList)
- val sorted = fibonacci() .skip(3). take (6). sortedByDescending() . toList()
- val sorted = fibonacci() .drop(3). take (6) .sortedDescending() .toList ()
5. What is the execution order of init blocks and properties during initialization?
- All of the properties are executed in order of appearance, and then the init blocks are executed.
- The init blocks and properties are executed in the same order they appear in the code.
- The order of execution is not guaranteed, so code should be written accordingly.
- All of the init blocks are executed in order of appearance, and then the properties are executed.
6. You want to know each time a class property is updated. If the new value is not within range, you want to stop the update. Which code snippet shows a built-in delegated property that can accomplish this?
- Delegates. cancellable()
- Delegates.watcher ()
- Delegates.observer()
- Delegates.vetoable()
7. You have a function simple () that is called frequently in your code. You place the inline prefix on the function. What effect does it have on the code?
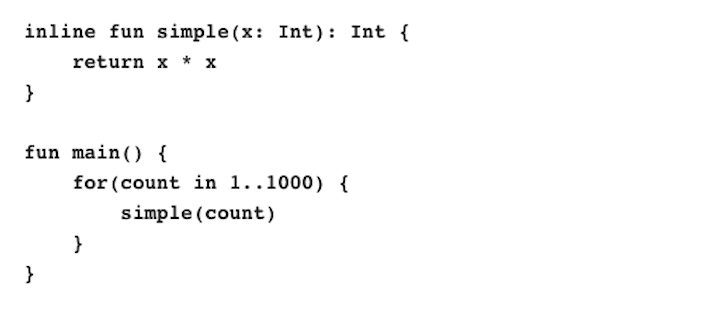
- The code will have a stack overflow error.
- The compiler warns of significant memory usage.
- The compiler warns of insignificant performance impact.
- The code is significantly faster.
8. Which line of code shows how to create a finite sequence of the numbers 1 to 99 and then converts it into a list?
9. Your application has an add function. How could you use its invoke method and display the results?
fun add (a: Int, b: Int): Int {
return a + b
}
- println(:: add.invoke(5, 10})
- println(add (5,10) . invoke () )
- println(add. invoke(5, 10))
- println(:: add.invoke (5, 10) )
10. What will happen when you try to build and run this code snippet?
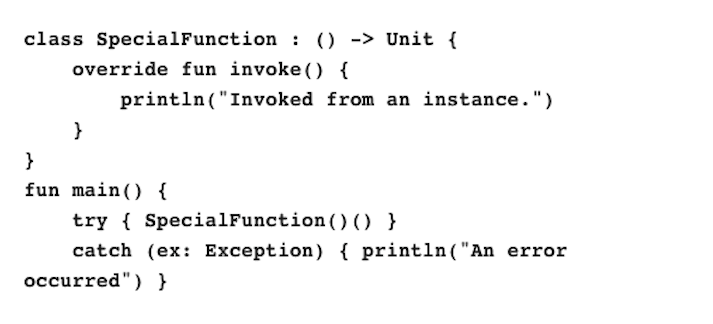
- The message “An error occurred” is displayed.
- The message “Invoked from an instance.” is displayed.
- A syntax error occurs due to the line SpecialFunction)0).
- A compile error occurs. You cannot override the invoke () method
11. You have created a data class, Point, that holds two properties, x and y, representing a point on a grid. You want to use the hash symbol for subtraction on the Point class, but the code as shown will not compile. How can you fix it?

- You should use minus instead of hash, then type alias the minus symbol.
- You need to replace operator with the word infix.
- You should replace the word hash with octothorpe, the actual name for the symbol.
- You cannot; the hash symbol is not a valid operator.
12. What are the two ways to make a coroutine's computation code cancellable?
- Call the checkCancelled() function or check the isCancelled property.
- Call the yield () function or check the isActive property.
- Call the stillActive() function or check the isCancelled property.
- Call the cancelled) function or check the isActive property.
13. Both y and z are immutable references pointing to fixed-size collections of the same four integers. Are there any differences?
val y = array0f (10, 20, 30, 40)
val z = listof (10, 20, 30, 40)
- You can modify the contents of the elements in y but not z.
- You add more elements to z since it is a list.
- You can modify the contents of the elements in 2 but not y.
- There are not any differences. x and y are a type alias of the same type.
14. When can you omit the constructor keyword from the primary constructor?
- It can be omitted anytime; it is not mandatory.
- It can be omitted when the primary constructor does not have any modifiers or annotations.
- It can be omitted if secondary constructors are defined.
- It can be omitted only if an init block is defined.
15. What is wrong with this class definition?
class Empty
- The class is properly defined, so nothing is wrong with it.
- The curly braces are missing from the declaration of Empty.
- The parentheses are missing-it should be declared as class Empty().
- Empty is a Kotlin keyword, so the code will generate an error when compiled.