automate cybersecurity tasks with python coursera weekly challenge 3 answers
Test your knowledge: Work with strings
1. Which of the following statements correctly describe strings? Select all that apply.
- Strings cannot contain numeric characters.
- Strings must be placed in brackets ([ ]).
- Strings must be placed in quotation marks (” “).
- Strings are immutable.
2. What does the following code return?
device_id = "uu0ktt0vwugjyf2"
print(device_id[2:5])
- “u0kt”
- “0ktt”
- “u0k”
- “0kt”
3. What does the following code display?
device_id = "Tj1C58Dakx"
print(device_id.lower())
- “tj1C58Dakx”
- “Tj1C58Dakx”
- “tj1c58dakx”
- “TJ1C58DAKX”
4. You want to find the index where the substring "192.168.243.140" starts within the string contained in the variable ip_addresses. Complete the Python code to find and display the starting index. (If you want to undo your changes to the code, you can click the Reset button.)
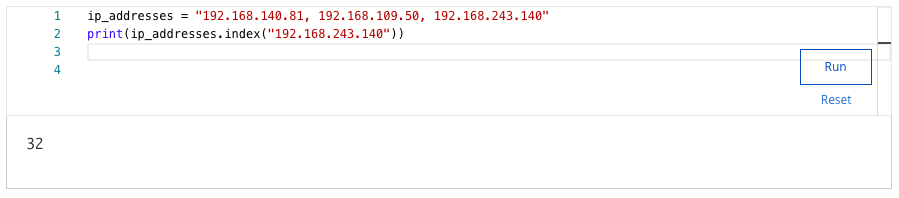
What index does the substring "192.168.243.140" start at?
- 31
- 33
- 34
- 32
Test your knowledge: Work with lists and develop algorithms
5. Review the following code:
my_list = ["a", "b", "c", "d"]
my_list[2] = 4
print(my_list)
What will it display?
- [“a”, 4, “c”, “d”]
- An error message
- [“a”, “b”, 4, “d”]
- [“a”, “b”, “4”, “d”]
6. You are working with the list ["cwvQSQ","QvPvX5","ISyT3a","S7vgN0"]. Its elements represent machine IDs, and the list is stored in a variable named machine_ids. Which line of code will add the ID of "yihhLL" at index 3?
- machine_ids.append(“yihhLL”,3)
- machine_ids.insert(“yihhLL”,3)
- machine_ids.append(“yihhLL”)
- machine_ids.insert(3,”yihhLL”)
7. Which line of code will remove the username "tshah" from the following list?
access_list = ["elarson", "bmoreno", "tshah", "sgilmore"]
- access_list[“tshah”].remove()
- access_list.remove(“tshah”)
- access_list.remove(2)
- access_list.remove(3)
8. As a security analyst, you are responsible for developing an algorithm that automates removing usernames that match specific criteria from an access list. What Python components would you help implement this? Select three answers.
- A for loop that iterates through the usernames in the access list
- The .remove() method
- The .append() method
- An if statement that compares a username to the criteria for removal
Shuffle Q/A 1
Test your knowledge: Regular expressions
9. Which regular expression symbol represents one or more occurrences of a specific character?
- \d
- \w
- *
- +
10. As a security analyst, you are responsible for finding employee IDs that end with the character and number sequence "a6v". Given that employee IDs consist of both numbers and alphabetic characters and are at least four characters long, which regular expression pattern would you use?
- “\w*a6v”
- “a6v”
- “\wa6v”
- “\w+a6v”