11. You have imported the re module into Python with the code import re. You want to use the findall() function to search through a string. Which function call enables you to search through the string contained in the variable text in order to return all matches to a regular expression stored in the variable pattern?
- findall(pattern, text)
- findall(text, pattern)
- re.findall(pattern, text)
- re.findall(text, pattern)
12. Which strings match the regular expression pattern "\w+"? Select all that apply.
- “3”
- “FirstName”
- “#name”
- “”
Weekly challenge 3
13. Which line of code converts the integer 7 to a string?
- str(“7”)
- str(7)
- string(7)
- string(“7”)
14. Which line of code returns a copy of the string "HG91AB2" as "hg91ab2"?
- print(“HG91AB2”.lower())
- print(“HG91AB2″(lower))
- print(lower.”HG91AB2″())
- print(lower(“HG91AB2”))
16. You need to take a slice from an employee ID. Specifically, you must extract the characters with indices of 3, 4, 5, and 6. Complete the Python code to take this slice and display it. (If you want to undo your changes to the code, you can click the Reset button.)
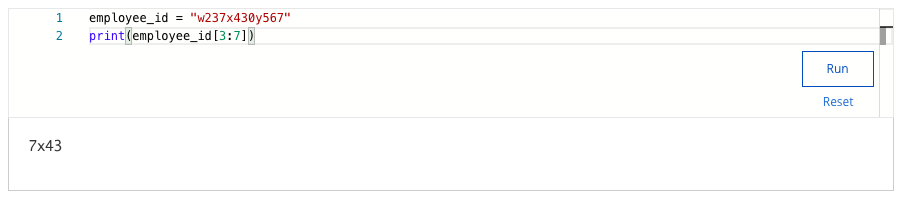
What string does the code output?
- “x430”
- “37×4”
- “7×43”
- “237x”
17. What is the output of the following code?
list1 = [1, 2, 3]
list2 = ["a", "b", "c"]
print(list1 + list2)
- [1, 2, 3, “a”, “b”, “c”]
- An error message
- [1, “a”, 2, “b”, 3, “c”]
- [6, “abc”]
18. A variable named my_list contains the list [1,2,3,4]. Which line of code adds the element 5 to the end of the list?
- my_list.insert(4,5)
- my_list.insert(5)
- my_list.insert(5,4)
- my_list.insert(5,5)
Shuffle Q/A 2
19. What is an algorithm?
- A function that finds matches to a pattern
- A set of guidelines to keep code consistent
- A function that returns information
- A set of rules to solve a problem
20. What does the \w symbol match to in a regular expression?
- Any letter
- Any character and symbol
- Any alphanumeric character
- Any number